Notice
Recent Posts
Recent Comments
Link
일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 |
Tags
- Navigation
- 기기고유값
- http
- NumberPIcker
- WorkManager
- log
- DialogFragment
- 화면 회전
- Room
- gradle plugin
- DataBinding
- Android
- Retrofit2
- BottomSheetDialogFragment
- ThreeTen Backport
- 생명주기
- layout_constrainedHeight
- todo
- multipart
- Lifecycle
- findNavController
- studywithme
- RecyclerView
- SSAID
- kotlin
- Load failed
- json
- Collections Function
- layout_constrainedWidth
- Popup menu background color
Archives
- Today
- Total
chacha's
💰 Chip 사용하기 본문
Kotlin Android Fundamentals: 10.3 Design for everyone
google-developer-training/android-kotlin-fundamentals-apps - Github
를 참고하여 작성하였습니다.
1. ChipGroup
생성하기
<HorizontalScrollView
android:id="@+id/chips"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintHorizontal_bias="0"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent">
<com.google.android.material.chip.ChipGroup
android:id="@+id/region_list"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:padding="16dp"
app:singleSelection="true"
app:singleLine="true"/>
</HorizontalScrollView>
2. ChipGroup 안에 사용할 Chip 레이아웃 생성하기
<com.google.android.material.chip.Chip xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
style="@style/Widget.MaterialComponents.Chip.Choice"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
app:checkedIconVisible="true"
tools:checked="true" // preview에서 체크된 상태를 보여주기 위해 사용
app:chipBackgroundColor="@color/selected_highlight"/>
app:chipBackgroundColor
는 chip이 체크되었을 때 백그라운드 색상을 바꿔주기 위해 사용합니다.
// res/color/selected_highlight.xml
<selector xmlns:android="http://schemas.android.com/apk/res/android">
<item android:color="?colorPrimaryVariant" android:state_selected="true" />
<item android:color="?colorOnSurface" android:alpha="0.18" /> // 82% 투명
</selector>
State List는 Top에서 Bottom으로 동작하므로 위와 같이 선언합니다. View의 상태(state)가 바뀌면 위의 코드가 Top에 위치한 코드부터 차례로 실행되면서 가장 먼저 매치되는 상태로 실행됩니다. 따라서 디폴트(default) 색상을 마지막(bottom)에 배치하면 다른 상태에서 매칭되지 못한 것들이 디폴트로 실행됩니다.
3. ChipGroup에 대한 Observer를 정의하고 Chips를 추가하기
viewModel.regionList.observe(viewLifecycleOwner, object: Observer<List<String>> {
override fun onChanged(data: List<String>?) {
data ?: return
// 1. list의 Item에 사용될 새로운 Chip View 만들기
val chipGroup = binding.regionList
val inflator = LayoutInflater.from(chipGroup.context)
// 2. listof<String>을 listof<View>로 생성
val children = data.map { regionName ->
val chip = inflator.inflate(R.layout.region, chipGroup, false) as Chip
chip.text = regionName
chip.tag = regionName // tag는 생성된 View에 넣을 아무 Object를 위한 공간
// onChanged Listener에서 tag는 선택된 chip의 regionName을 가져올 때 사용합니다.
chip.setOnCheckedChangeListener { button, isChecked ->
viewModel.onFilterChanged(button.tag as String, isChecked)
}
chip // 새로운 리스트 반환
}
// 3. chipGroup에 이미 존재하는 view를 제거
chipGroup.removeAllViews()
// 4. ChipGroup에 새로운 view children을 추가
for (chip in children) {
chipGroup.addView(chip)
}
}
})
실행 결과
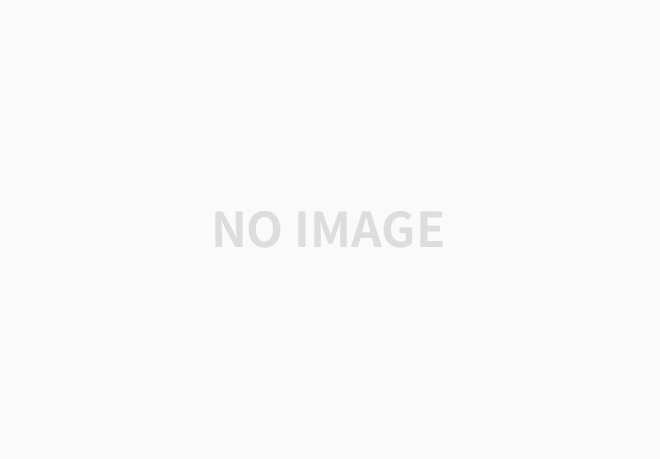
'Android > My Library' 카테고리의 다른 글
💬 Popup Menu 사용하기 (0) | 2021.11.23 |
---|---|
⏰ Android FCM 푸시 알림 (0) | 2021.11.08 |
🧭 Navigation을 이용해서 Dialog로 전환하기 (0) | 2021.06.09 |
♻ RecyclerView 에 🎩Header 추가하기 (0) | 2021.06.07 |
♻ RecyclerView에 👆 Click 이벤트 추가하기 (0) | 2021.06.07 |